[vb6.0vba] [VB .NET] Multi-Threading and Delegates Tutorial in VB .NET
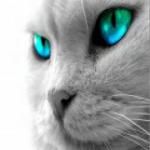
본문
This guide will show you everything you need to create a non-GUI multi-threaded application in VB .NET.(이 안내서는 VB.NET에서 GUI가 아닌 멀티 스레드 응용 프로그램을 만드는 데 필요한 모든 것을 보여줍니다.)
There are 2 ways of using multi-threading in VB .NET. First by making use of delegates which I will explain here. And second by programming the threads manually (not actually more complicated).(VB.NET에서 멀티 스레드를 사용하는 두 가지 방법이 있습니다. 첫 번째는 여기서 설명할 대리인을 활용하는 것입니다. 두 번째는 스레드를 수동으로 프로그래밍하는 것입니다(사실 더 복잡하지는 않습니다).)
Using delegates, .NET helps simplify the entire process of using a separate thread and saves you from having to manage the parameter passing, result retrieving and timing issues.(딜러를 사용하여 .NET은 별도의 스레드를 사용하는 전체 프로세스를 간소화하고 매개 변수 전달, 결과 검색 및 타이밍 문제를 관리할 필요가 없도록 지원합니다.)
First to simply call a method on a separate thread with a parameter(먼저 매개 변수를 사용하여 별도의 스레드에서 메서드를 간단하게 호출합니다)
Delegate Function MyDelegate(ByVal text as String) as StringFunction PrintStuff(ByVal text as String) as String Console.WriteLine(text) Thread.Sleep(5000) Return "Success"End FunctionSub Main() Dim d as MyDelegate = AddressOf PrintStuff d.BeginInvoke("hello world", Nothing, Nothing) 'I can do more stuff hereEnd Sub
Here we have a Main thread and a second thread to print some stuff. Also, a delegate with the same signature as the function we want to run (PrintStuff) already exists. Very simply, the Main thread creates a delegate that points to PrintsStuff and calls for the delegate to start executing PrintStuff on a separate thread. A parameter is passed to PrintStuff in the process and Main can continue working while PrintStuff is busy.Now suppose you want to know when PrintStuff finishes because you want the result "Success". We can do this by the use of a callback function.First, we create the callback function(여기에 일부를 인쇄하기 위한 메인 스레드와 두 번째 스레드가 있습니다. 또한 실행하려는 기능(PrintStuff)과 동일한 서명을 가진 위임자가 이미 있습니다. 매우 간단하게 메인 스레드는 PrintStuff를 가리키는 위임자를 만들고 위임자가 별도의 스레드에서 PrintStuff 실행을 시작하도록 요청합니다. 매개 변수는 프로세스에서 PrintStuff에 전달되고 PrintStuff가 바쁜 동안 메인 작업을 계속할 수 있습니다. 이제 "성공"이라는 결과를 원하기 때문에 PrintStuff가 언제 끝나는지 알고 싶다고 가정해 보겠습니다. 콜백 함수를 사용하여 이 작업을 수행할 수 있습니다. 먼저 콜백 함수를 만듭니다)Sub MyCallback(ByVal result as IAsyncResult) Console.WriteLine("Now I know PrintStuff finished")End SubThen we make sure the callback function gets called by changing the previous BeginInvoke command to(그런 다음 이전의 BeginInvoke 명령을 다음과 같이 변경하여 콜백 기능이 호출되는지 확인합니다)d.BeginInvoke("hello world", New AsyncCallback(AddressOf MyCallback), Nothing)In other words, the before last parameter (depending on how many parameters your MyDelegate is supposed to take, since they come in front) is where you specify your callback function. Now MyCallback will be called when PrintStuff is done (ie after about 5 seconds)Now we need MyCallback function to actually read the return of PrintStuff ("Success"). It is contained in the IAsyncResult.(즉, 이전 마지막 매개 변수(내 대리인이 몇 개의 매개 변수를 취해야 하는지에 따라 앞에 오기 때문에)는 콜백 기능을 지정하는 위치입니다. 이제 PrintStuff가 완료되면(즉, 약 5초 후) MyCallback이 호출됩니다 이제 PrintStuff("성공")의 반환을 실제로 읽으려면 MyCallback 함수가 필요합니다. IAsyncResult에 포함되어 있습니다.)Sub MyCallback(ByVal result as IAsyncResult) Console.WriteLine("Now I know PrintStuff finished") Dim resultClass = CType(result, AsyncResult) Dim d as MyDelegate = CType(resultClass.AsyncDelegate, MyDelegate) Console.WriteLine("And I also know that the result is: " & d.EndInvoke(result))End SubIn other words, (instance of MyDelegate).EndInvoke will give you the return of PrintStuff. You could have simply called it in your Main instead of MyCallback, but then Main will remain on that line until PrintStuff reaches its Return line, defeating the whole purpose of multi-threading. When you use EndInvoke in the callback function, you are guaranteed that PrintStuff has already reached its Return line and you simply have to retrieve the result.For multi-threading applications involving GUI elements, check by other tutorial to see how to avoid illegal cross-thread operations.(즉, ,(MyDelegate.EndInvoke)는 PrintStuff를 반환합니다. 단순히 MyCallback 대신 Main에서 호출할 수 있었지만, PrintStuff가 Return 행에 도달할 때까지 Main은 해당 행에 남아 있어 멀티 스레드의 전체 목적을 충족시킵니다. 콜백 기능에서 EndInvoke를 사용하면 PrintStuff가 이미 Return 행에 도달했음을 보장하고 결과를 검색하기만 하면 됩니다. GUI 요소가 포함된 멀티 스레드 응용 프로그램의 경우 다른 튜토리얼을 통해 불법적인 크로스 스레드 작업을 방지하는 방법을 확인하십시오.)
댓글목록0